In other languages simple things are easy and complex things are possible, in Rust simple things are possible and complex things are EASY.
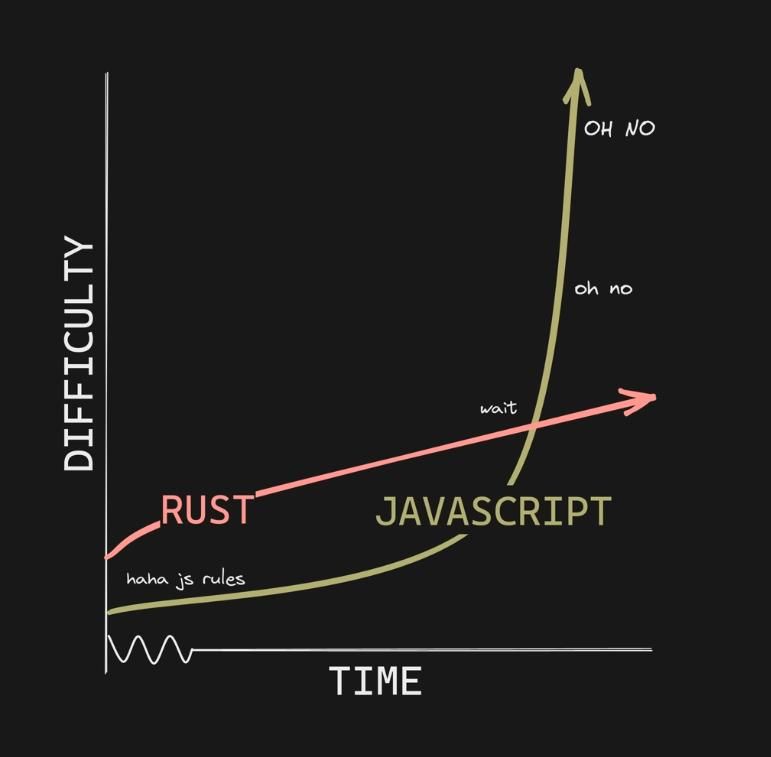
Rust is a programming language and a general-purpose, systems-programming platform. Rust is a memory safe language which means that it prevents you from accidentally corrupting your data or executing malicious code. Rust uses a “systems programming” approach to programming, focusing on safety over speed and efficiency. This means that you need to think about the big picture when writing code, rather than just worrying about how many milliseconds it takes to run your function.
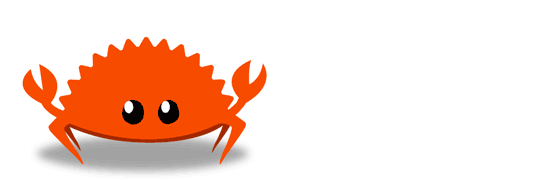
Rust is designed to be a systems programming language, which means that it can be used to create applications that run on a wide range of platforms. Rust also has strong support for concurrency and parallelism which makes it especially suited to writing high performance library code.
Rust has been Stack Overflow’s most liked programming language for four years in a row, indicating that many of those who have had the opportunity to use Rust fallen in deeper relation with it.
Substrate is a blockchain development framework with a completely generic State Transition Function STF and modular components for consensus, networking, and configuration.
Despite being “completely generic”, it comes with both standards and conventions - particularly with the Substrate runtime module library (a.k.a FRAME) - regarding the underlying data-structures that power the STF, thereby making rapid blockchain development a reality.
Architecture of Substrate framework:
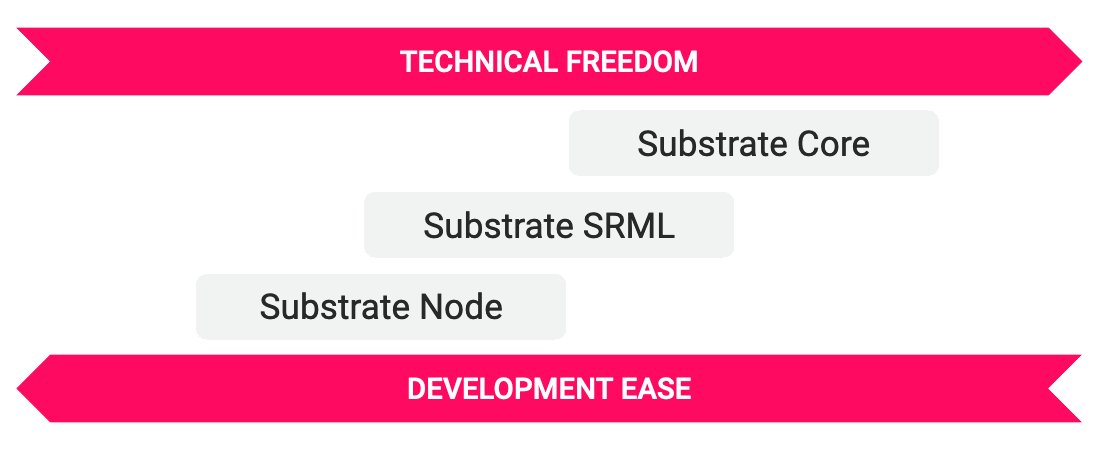
What Substrate delivers - the game changer
Be honest. Delivering distributed systems is complex and hard, many companies try to deliver usable solution, often spend millions of dollar, not rare hire 10x software engineers and pays them really a lot but still deliver usable solution is hard.
In my opinion Substrate and writing own parachain for Kusama Network is on the way to make simpler design and implement really usable solution. Why?
Because being a parachain removes following difficulties:
Security of given network
Proof of work, consensus of a network
Runtime upgrades - forget about forks (!!!)
Patterns in The Environment
Rust Syntax Patterns
0. Macro
Macro is a code which expands already existing source code:
1/// main developer interface of Substrate pallet2decl_storage! {3 /// storage data on-chain4 trait Store for Module<T: Trait> as Kitties {5 /// Stores all the kitties, key is the kitty id6 pub Kitties get(fn kitties): double_map hasher(blake2_128_concat) T::AccountId, hasher(blake2_128_concat) T::KittyIndex => Option<Kitty>;7 /// Stores the next kitty ID8 pub NextKittyId get(fn next_kitty_id): T::KittyIndex;9 }10}1112decl_module! {13 pub struct Module<T: Config> for enum Call where origin: T::Origin {1415 }16}1718decl_event! {19 pub enum Event {2021 }22}
1. Turbofish
1impl<T: Trait> Module<T> {2 fn update_storage(transaction: &Transaction) -> DispatchResult {3 for input in &transaction.inputs {4 /// turbofish syntax5 <UtxoStore>::remove(input.outpoint);6 /// end of turbofish7 }89 Ok(())10 }11}
2. Closure
1Utxo build(|config: &GenesisConfig| {2 config.genesis_utxos3 .iter()4 .cloned()5 .map(|u| (BlakeTwo256::hash_of(&u), u) )6 .collect::<Vec<_>>()7}): map hasher(identity) H256 => Option<TransactionOutput>;
3. Option
syntax
Option
is a type of Enum
that either returns Some
with the variable inside or returns an enumerator None
and it is a good practice to encapsulate code in an Option
.
1UtxoStore: map H256 => Option<TransactionOutput>; //example
4. DispatchResult as Error Handling
1pub fn spend(_origin, transaction: Transaction) -> DispatchResult {2 // ...3 Ok(()) // or Err4}
Blockchain and Substrate Design Patterns
1. UTXO Transaction Input Output
1pub struct TransactionInput {2 pub outpoint: H256, // reference to a UTXO to be spent3 pub sigscript: H512,// proof4}56pub struct TransactionOutput {7 pub value: Value, // value associated with this UTXO8 pub pubkey: H256, // public key associated with this output, key of the UTXO's owner9}1011pub struct Transaction {12 pub inputs: Vec<TransactionInput>,13 pub outputs: Vec<TransactionOutput>,14}
2. Configuration of genesis state in Substrate (useful for testing)
1decl_storage! {2 trait Store for Module<T: Trait> as Utxo {3 Utxo build(|config: &GenesisConfig| {4 config.genesis_utxos5 .iter()6 .cloned()7 .map(|u| (BlakeTwo256::hash_of(&u), u) )8 .collect::<Vec<_>>()9 }): map hasher(identity) H256 => Option<TransactionOutput>;10 }1112 add_extra_genesis {13 config(genesis_utxos): Vec<TransactionOutputs>;14 }15}
My notebook of Joshy’s and Dan’s Seminars
An initial assumption of the post is to share my thoughts about Substrate and Rust but also to create a notebook for snippets from so called “Joshy’s Seminars” which are great! Thanks Joshy!
;-)
The seminars previously was recorded via Zoom and here is the link to YouTube currently are available via Crowdcast.
15-09-2020
https://hackmd.io/Msx2ZYb5QX2FxSWPdIpwgg?view
https://substrate.dev/recipes/kitchen-node.html
06-08-2020
1https://polkadot.js.org/apps/#/settings?rpc=ws://127.0.0.1:9944
28-07-2020: Substrate Enterprise Demo
Role-based Access Control Pallet https://github.com/gautamdhameja/substrate-rbac
Decentralized Identifiers Pallet https://github.com/substrate-developer-hub/pallet-did
Product Tracking Pallet https://github.com/stiiifff/pallet-product-tracking
Product Registry Pallet https://github.com/stiiifff/pallet-product-registry
Validator Set Pallet https://github.com/gautamdhameja/substrate-validator-set
26-05-2020
- https://github.com/paritytech/substrate-subxt
- https://safecurves.cr.yp.to/index.html
- https://github.com/hammeWang/substrate-sm2
- https://crates.parity.io/sp_runtime/enum.MultiSignature.html
- https://stackoverflow.com/questions/454048/what-is-the-difference-between-encrypting-and-signing-in-asymmetric-encryption
- https://crypto.stackexchange.com/questions/9918/reasons-for-chinese-sm2-digital-signature-algorithm
- https://andrea.corbellini.name/2015/05/17/elliptic-curve-cryptography-a-gentle-introduction/
- https://en.wikipedia.org/wiki/Edwards_curve
- https://blog.cloudflare.com/a-relatively-easy-to-understand-primer-on-elliptic-curve-cryptography/
- https://gitlab.com/totem-tech/totem-substrate/-/blob/master/node/runtime/src/boxkeys.rs
- YouTube: [TUTORIAL] Build a Bitcoin-like Blockchain with Substrate - Beginner Friendly (1 of 5)
Links
https://medium.com/paritytech/why-rust-846fd3320d3f
https://substrate.dev/docs/en/knowledgebase/integrate/memory-profiling
https://github.com/w3f/consensus/blob/master/pdf/grandpa-old.pdf
https://wiki.polkadot.network/docs/en/learn-consensus#grandpa-finality-gadget
https://marketplace-staging.substrate.dev/
https://www.forrestthewoods.com/blog/how-to-debug-rust-with-visual-studio-code/
https://hackmd.io/OzLUIGA8QQC3Ketgsd7vqg
https://github.com/paritytech/substrate-debug-kit
https://substrate.dev/en/seminar
https://blog.chain.link/44-ways-to-enhance-your-smart-contract-with-chainlink/
https://github.com/smartcontractkit/chainlink-polkadot
Rust courses
. . to be continued . .
Coffee time
Dear Reader, if you think the article is valuable to you and you want me to drink (and keep writing) high quality coffee (I do like it), feel free to buy me a cup of coffee. ⚡